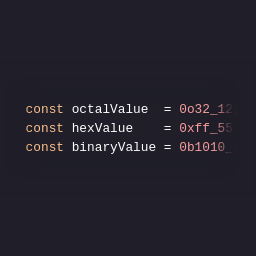
What are numeric separators?
2021-03-11
When working with large numbers it can be hard to read them out, try to read this value for example: …read more
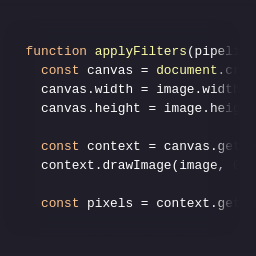
JavaScript array methods and how to use them
2021-03-06
Arrays are as central to JavaScript as in many other languages, in this article we will go through the most useful array methods along with creative usage examples. …read more
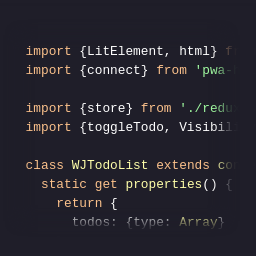
How to build a Web Components app with Redux!
2021-03-01
The flux pattern has proved useful for more complex applications. The Redux npm package has been used extensively in React applications for years but can also be used in similar ways with LitElement style web components. …read more
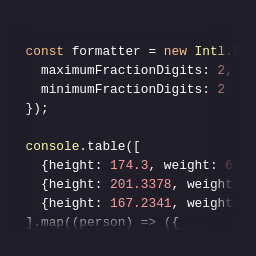
How to format numbers
2021-02-28
The native Intl.NumberFormat API lets you format numbers for specific languages without any external dependencies. …read more
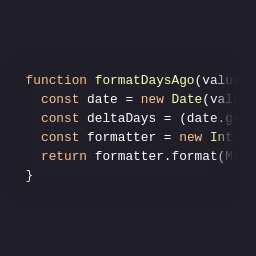
Format "5 days ago" localized relative date strings in a few lines with native JavaScript
2021-02-27
The native Intl.RelativeTimeFormat API can generate nicely formatted relative date/time strings without any external dependencies. …read more
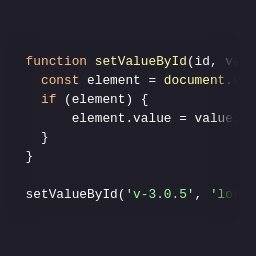
Escaping your CSS selectors in the easiest way possible
2021-02-25
When working a lot on dynamic Web Component apps I have often stumbled on cases where the element properties will be defined by the end-users. To prevent errors when selecting these elements they need to be properly escaped to work with any character. …read more
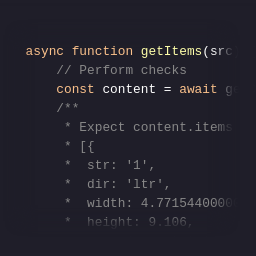
How to extract pdf data with PDF.js
2021-02-24
We live in a data-driven world, consistently transferring data from one location to another. In this brief tutorial, I will show you how to extract pdf content using PDF.js. This npm package will help you roll out custom pdf extraction logic or an interface to explore pdf data. …read more
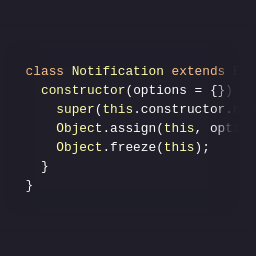
Use more ergonomic custom events
2020-09-21
While we have CustomEvent for all of our events that need to carry data the need for the detail property nesting can feel a bit cumbersome. …read more
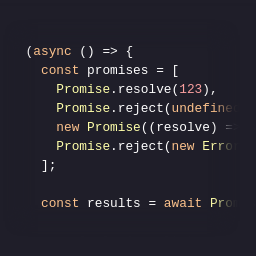
10 great JavaScript tricks that will improve your productivity
2020-09-21
These top tips and tricks will help you dramatically increase your productivity while coding in any JavaScript project. …read more
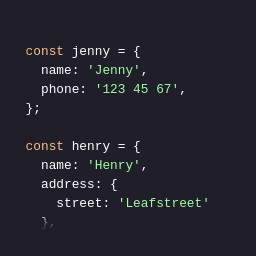
Why should I start using Optional Chaining and Nullish Coalescing operators?
2020-09-20
Gone are the days where you had to add incremental nullish (undefined or null) checks when fetching values in nested objects. Let's do more with less code. …read more
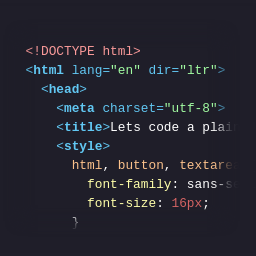
Lets code a plain JavaScript notification queue using private fields and methods
2020-09-18
Defining easy-to-use APIs can be tricky, a good starting point is to keep a small exposed surface. Now as private fields are becoming available, let try them out. …read more
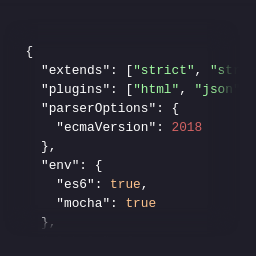
ESLint disable single line and code blocks
2020-09-16
ESLint is a must-have tool for the editor as well as in your CI setup as it greatly improves code quality, however, sometimes you need to disable some rules. …read more
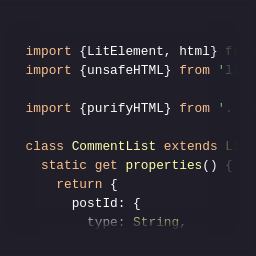
Keep your HTML output secure and clean from XSS JavaScript injection
2020-09-15
Writing secure web services can be hard, several attack vectors exist, this article explains how XSS or JavaScript injection can be prevented. …read more
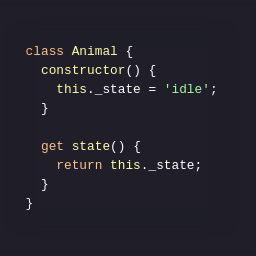
How-to create and use mixins in JavaScript
2020-09-15
Mixins are handy when you want to share a feature across multiple classes without using inheritance as only some of the child classes should have that feature. …read more
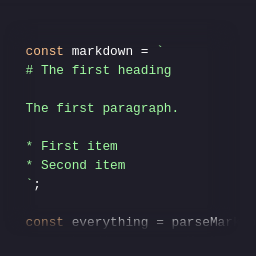
How to use keyword arguments in JavaScript
2020-09-15
Passing an Object named options to a function instead of separate arguments is one of the oldest tricks in the book, by also assigning an empty object by default you also reduce the amount of if statements needed at the top of the function. But a year ago I learned how combining this with object deconstruction made it so much more elegant. …read more
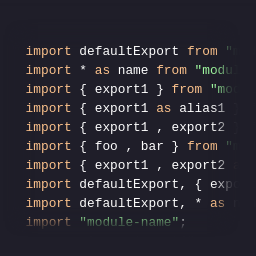
Why default exports are bad
2020-09-15
Import statements come in a lot of different flavors in JavaScript and where there is variation some of the options are often better than others. For import statements, we want to easily understand which parts of a module we are going to use and also easily get an overview of where those parts are being used throughout our projects. …read more
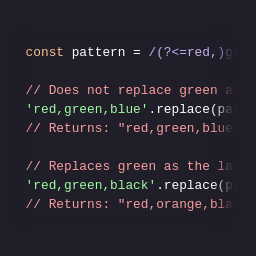
How to use lookahead and lookbehind RegExp in JavaScript
2020-09-15
Sometimes you want to ensure that a RegExp pattern starts immediately after a specific string but you don't want to include those characters in the match. In these cases, a lookbehind expression comes in handy. …read more
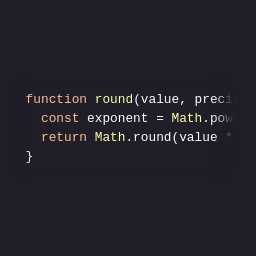
Rounding a number to a specific precision in JavaScript
2020-09-13
This is a tiny and handy function to round a number to a specific precision: …read more
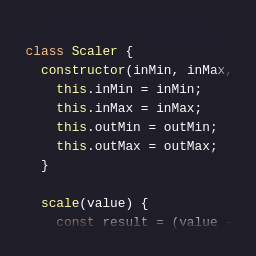
Scaling values between two ranges
2020-09-13
In some cases, you have one type of value with a specific range and you want to scale them proportionally into a new range. …read more
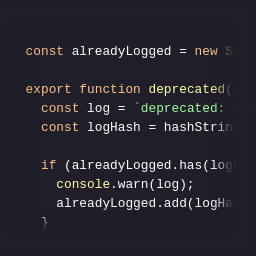
How to deprecate features in your API before making breaking changes
2020-09-13
When creating APIs that external parties depend on you will soon realize the need to be careful about making breaking changes to that API as even a single renamed property/function might cause existing code using your API to fail. …read more
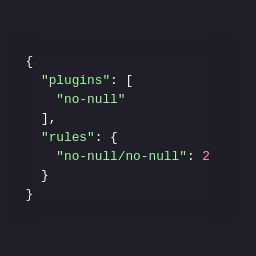
Why you should always use undefined, and never null
2020-09-12
To write simple code you need to keep complexity and variation down. I find that where JavaScript offers alternatives, stick to one of them. …read more